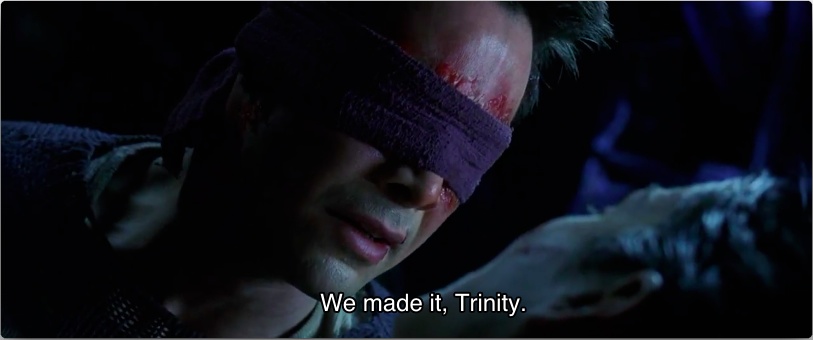
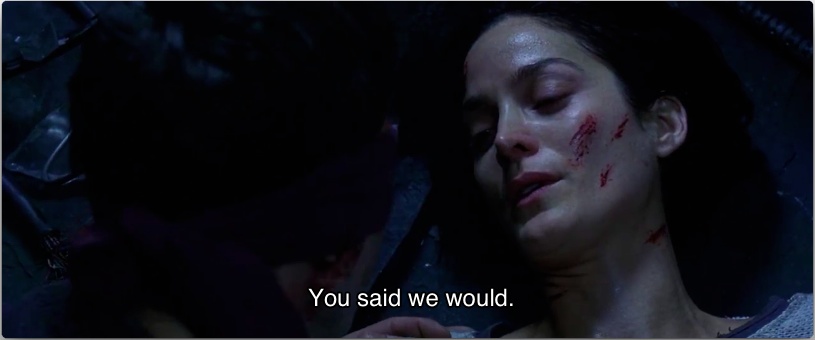
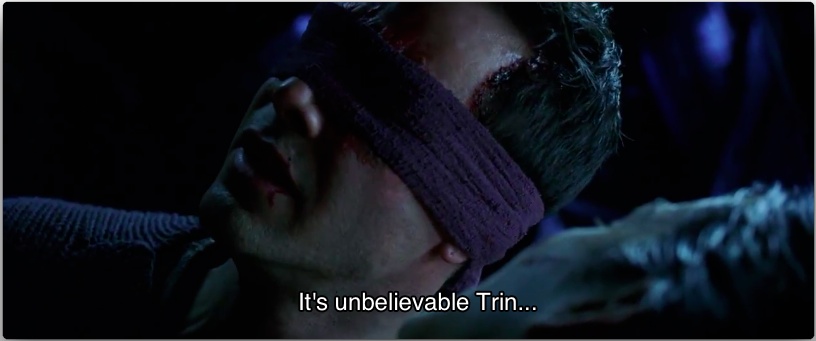
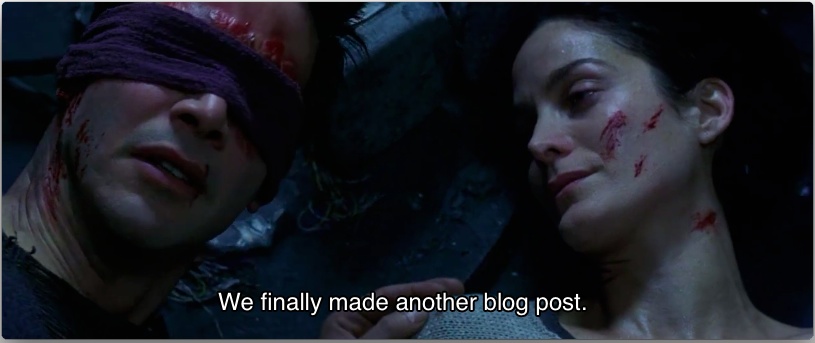
Agenda
I want to talk about iOS app architecture and Test Driven Development.
For the past couple of years I’ve been developing the feeling that there’s something seriously wrong with how a lot of people are approaching iOS development. Apple has made certain decisions with their framework design and tools which I believe contributes to this problem.
Specifically I’m talking about where the application and business logic for an app belongs, and how the overall architecture of an app should be constructed. I feel I need to slap some people across the face and bring them to their senses (including myself).
This is going to be the first in a series of posts. Today we’re going to find out why Apple has created a total abomination of design with UIViewController
. Should be fun.
In future posts I will describe an Uncle Bob style “Clean Architecture” for iOS apps, driven by TDD. I haven’t seen anything like this from other writers/developers, so be sure to subscribe (or follow me on twitter) if that sounds interesting.
The Humble View Controller?
Way back in 2002, Michael Feathers (of “Working Effectively with Legacy Code” fame) wrote a piece titled The Humble Dialog Box. In it, he describes a particular approach to separating GUI-specific code from the actual application logic behind a simple dialog box.
We’ll revisit this piece sometime in the future, but for now I want to focus on the introductory problems he describes. Remember he is talking about building desktop applications here:
Let’s take a look at our friend the dialog box. […]
Tool vendors have made it easy to create dialog boxes. Nearly every IDE on the market has a GUI builder. You can drag and drop all sorts of components on dialogs and drill down to wire them up. […]
It is easy to just override an event from a component and drop your interaction logic right there in the dialog box class.
He goes on to describe the problems that typically arise with these dialog box classes:
- It’s difficult to get these UI classes under a test harness because they are so tied to UI-specific frameworks. There is no separation between the UI and interaction/application logic.
- The UI classes have a mixture of low level component API code as well as actual decision making code, making it hard to see what the code is doing or to share code between objects with similar behaviour.
These statements perfectly describe a typical iOS view controller. It’s easy to drop code into an IBAction
method or a delegate call on the view controller, and do everything right there and then. This can lead to a mixture of “component API” (i.e. UIKit) and important decision making code.
Apple has made UIViewController
the center of the iOS universe. When you add a new screen to your app, the only thing that Xcode gives you is a new UIViewController
subclass. And Apple makes it so easy to do everything from this view controller. If you aren’t really careful, you can end up with an application consisting entirely of view controller classes and little else.
Now of course there are good developers out there who are creating nicely decoupled classes, but if we list out some of the responsibilities that a view controller typically ends up dealing with, we’ll see just how pervasive this problem is, even in code written by experienced developers.
Spotted in the wild
View setup and layout code
By default, when you hook up a new control to your view controller’s XIB or storyboard (for example a UIButton
or UILabel
), the IBOutlet connection is going to go to a property on your view controller. The whole assistant-editor workflow is designed to do this. All of the sample code, tutorials and books you will ever read will do this as well.
If you need to tweak that control’s settings in code, your first stop will probably be the trusty -viewDidLoad
method:
- (void)viewDidLoad { [super viewDidLoad]; self.myButton.titleLabel.font = myCustomFont; UIImage *stretchableImage = /* ... blurgh ... */ [self.myButton setBackgroundImage:stretchableImage forControlState:UIControlStateNormal]; /* ... more 'blurgh' ... */ }
Over time, your -viewDidLoad
will likely grow. You may even create new controls in here that don’t exist in your XIB file. Even if you break things out into multiple methods, a significant part of your view controller’s code can end up dealing with view setup and creation. The situation is worse if you forgo XIB files entirely.
And what if you have custom layout requirements which can’t be solved in IB?
Well… you could create a UIView
subclass and implement -layoutSubviews
. But you already have all your IBOutlets going to the view controller. You would have to move all the IBOutlet connections and properties to this new view class — and why bother with all that when it’s so easy to add some code to -viewWillAppear:
:
- (void)viewWillAppear:(BOOL)animated { [super viewWillAppear:animated]; self.myLabel.frame = /* ... */ self.myButton.frame = /* ... */ }
Even though using a UIView
subclass and overriding -layoutSubviews
is a far superior way to layout your controls (and is in fact the correct way), people end up putting this stuff in their view controllers because that’s the easiest thing to do.
And then they have to deal with view rotation issues, and not being told when the view’s frame changes. Even worse, because this stuff is so hard to do properly from the view controller, people often end up hard coding interface sizes and frames rather than implementing truly dynamic view layouts.
I’ve seen people doing layout in the -viewDidLoad
method, before the view is even part of the view hierarchy. And they wonder why things mess up. You can’t even blame them. After all, it is Apple who is encouraging people to connect their IBOutlets to the view controller.
It all becomes a hideous mess, and could easily be solved with UIView
subclasses. But Apple doesn’t teach us to do this. I doubt many novice or intermediate iOS developers have even heard of -layoutSubviews
or -sizeThatFits:
. All Apple gives us by default is a view controller subclass.
Even with Autolayout the problem doesn’t go away. The view controller still ends up being the place to create custom constraints, for the same reasons as above. That’s just how people do it. Even Apple engineers do it this way in their WWDC videos.
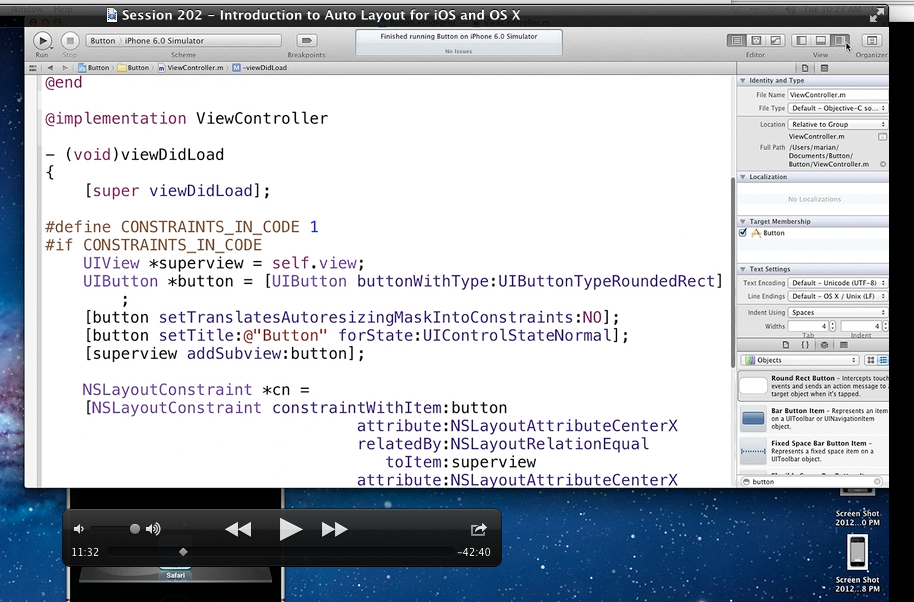
So the Xcode/Apple-approved default is to have all of your view setup and layout code in the view controller. Why is this a bad thing? Quite simply, because view layout and setup code should (in my opinion) always go in UIView
subclasses. That’s where it belongs, and life is a lot easier when you do it this way. Putting this stuff in the view controller is just contributing to an already bloated and confused class.
Core Data and NSFetchedResultsController
code
All of the Core Data sample code that I’ve seen from Apple creates and sets up the NSFetchedResultsController
in a -fetchedResultsController
method on the view controller. It’s usually a huge long method which sets up the fetch request, configures the fetchBatchSize
, predicate, sort descriptors etc.
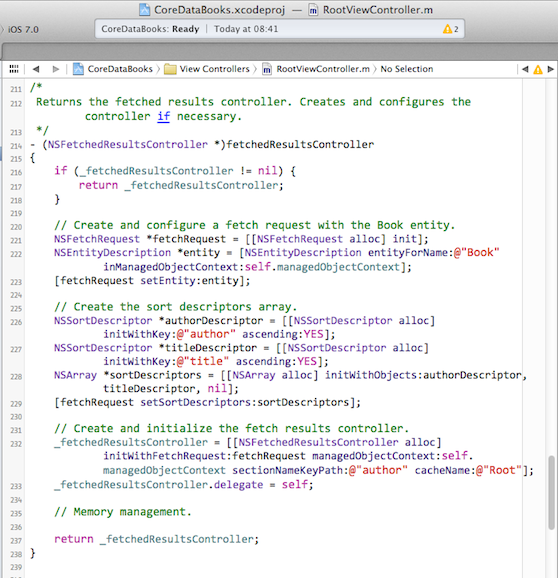
Unfortunately I’ve seen a lot of people who have copy/pasted this kind of code into their view controllers. As time goes on, the view controller ends up with a dozen methods just dealing with Core Data - fetching results, updating records, performing background operations, merging contexts, etc.
None of this stuff belongs in the view controller. You have business logic like sorting and filtering rules mixed in with the details of the persistence framework (Core Data), and all of this inside the view controller. A “view controller” should not be dealing with this stuff, and Apple unfortunately does not show new-comers how to do this properly.
Be the delegate for everything
Even if you pull your Core Data code out into a nice class or interface, your view controller will probably end up being the delegate and datasource for the table view displaying that data. It’ll contain the code to create and configure table view cells for a given item, define section titles and row heights, handle insertion and deletion operations, and respond to any of the dozen or more delegate/datasource callbacks that are available.
If your app is making use of CLLocationManager
, your view controller will probably end up responding to and handling location events. It might even be the one that creates the location manager in the first place. Another of Apple’s tutorials does just this.
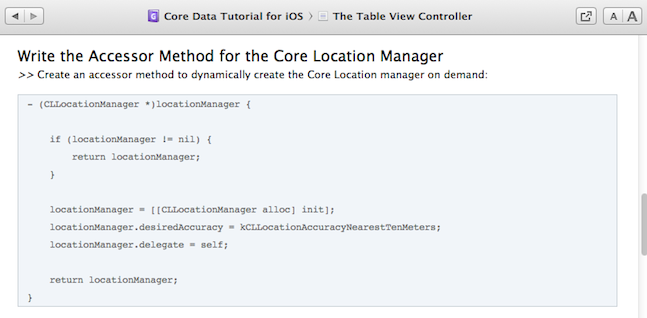
If your view controller makes use of MPMoviePlayerController
or QLPreviewController
or MFMailComposeViewController
you’ll probably be the delegate for those as well. You can also throw in Twitter and Facebook API callbacks (and sharing in general), quick one-off AFNetworking
or NSURLConnection
web service requests, NSTimer
s doing periodic background work, SCNetworkReachability
checks, and the list goes on.
Because UIViewController
s are such a central part of an iOS app, they end up being the delegate for everything. They end up accumulating all this crap. If the view controller was purely forwarding information and acting as an intermediary, this might not be so bad. But we all know that’s rarely the case.
You really have to be on the ball to keep this stuff nicely separated, and I’ve seen a lot of codebases which fail to do this.
Accessing global state
Because it’s so easy to drop code into a view controller, and because Xcode doesn’t give you any other place to start adding your code, inexperienced developers have trouble accessing the information they need.
They end up using hideous hacks like ((IHaveNoIdeaWhatImDoing*)[[UIApplication sharedApplicaton] delegate]).objectThatINeed
, or singletons, or notifications, to access everything they need. Stackoverflow and internet forums get inundated with questions of the form “How to I pass information from one view controller to another”?
The reason this is so difficult is that they haven’t been taught to write higher-level objects which deal with the interactions and flow of information in their app.
So not only do many view controllers have dozens of delegate calls coming in to them, they also have hideous clawed tentacles reaching out all over the application, accessing global state and further coupling the view controller to other parts of the application.
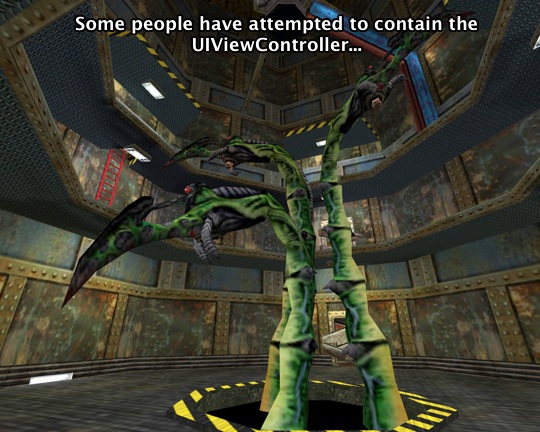
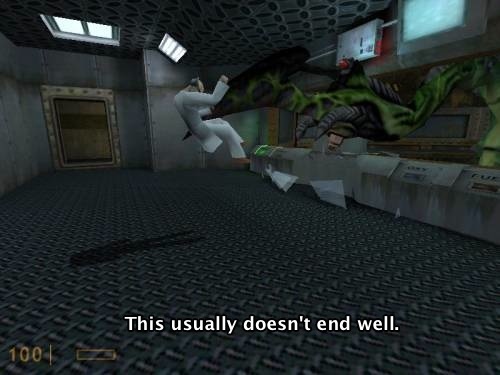
You can argue that this is just a problem of inexperienced developers, but Apple really does not help people to develop good coding practices. I’ve seen Apple sample code using [[UIApplication sharedApplicaton] delegate]
to access global state, and it’s really a terrible practice to encourage. In fact I already have a blog post half-written which deals precisely with this topic.
Navigation logic and other “decision making” code
When the user selects a cell from your table view, you are going to handle the tableView:didSelectRowAtIndexPath:
method.
Your app now has to decide what to do. In a typical drill-down style of navigation, you want to push some sort of “details” screen for the selected item.
Apple makes this really easy with convenience methods on UIViewController
like -navigationController
, -tabBarController
, -splitViewController
, etc. Your view controller can directly grab a reference to the container that holds it.
You can then do something like this:
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath { id item = [self itemAtIndexPath:indexPath]; DetailsViewController *detailsVC = [[DetailsViewController alloc] initWithItem:item]; [self.navigationController pushViewController:detailsVC animated:YES]; }
Perfect! The navigation logic can be dropped right there in the view controller!
But what if you later make your app universal, and the details screen needs to appear in a split view controller on iPad devices?
Well, no problem. We’ll add a little runtime check:
DetailsViewController *detailsVC = /* ... */; if (UI_USER_INTERFACE_IDIOM() == UIUserInterfaceIdiomPad) { // display details in the split view controller's details pane } else { // push details to the current navigation controller }
We also need to keep the cell selected on the iPad, but deselect it in viewWillAppear:
on iPhone devices (this is what UITableViewController
does by default). This adds a few more if statements to the controller.
But what if you now want to present this view controller modally at some point in your application so the user can select one item for a task? In that case you need to dismiss the view controller and somehow notify the parent about which item was selected.
We can’t keep adding more and more if statements to our view controller. It’s pretty clear that this kind of navigation logic doesn’t belong in the view controller.
A view controller should not know about the high level work flow of your app. It shouldn’t know about its surroundings. It shouldn’t know that it’s being presented modally, or that it’s contained by a navigation controller, or a split view controller, or a tab bar controller. It shouldn’t even have references to these things, even though Apple decided to “help” us out by tacking on all these category methods. It shouldn’t even know about the DetailsViewController
. That’s an entirely different screen of our app.
If our view controller is displaying a list of items (think a typical table view controller), that’s all it should be concerned with. It should provide some delegate callbacks for when a given cell or item is selected, and perhaps a few ways to customize the display or behaviour of the cells, for example to enable a check-multiple-items style of selection.
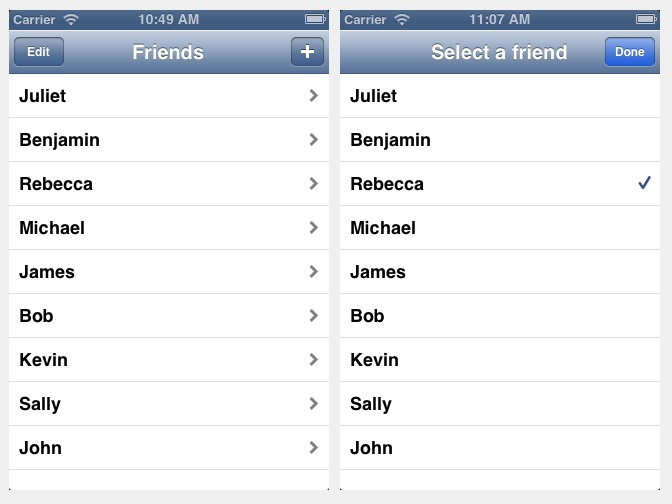
The view controller should only be concerned with the behaviour of the view that it presents. By putting navigation logic in the view controller, you are coupling it to the specifics of your app’s UI and navigation. If you want to change the workflow later (e.g. for other devices), or reuse that view controller in a different context (or even a different app), you are going to encounter a lot of pain.
So where should this navigation logic go? Well… somewhere else. We’ll get into that in a later post. All that matters for now is that the view controller should delegate UI actions like this to somebody at a higher level, who has access to and knowledge of the specifics of your app’s UI workflow.
This is also why I have a big problem with Storyboards. When you implement a Storyboard app, you are forced to put application-specific navigation logic in the view controllers by implementing the various UIViewController
segue-related methods. There’s no other way to do it. And delegating this stuff out to somewhere else really confuses the matter because you have this extra level of indirection between the storyboard XIB and the segue code. Reusing view controllers in other apps or screens suddenly becomes impossible to do (or at least very difficult) without creating a total mess of things.
Official UIKit responsibilities
So far we’ve mostly looked at logic and code that could theoretically be pulled out of the view controller and put somewhere else. You’ll probably be doing this already to a greater or lesser extent.
But what about the stuff that a UIViewController
must deal with? What about it’s official UIKit responsibilities?
In fact, what the hell is a “view controller” anyway? Does anybody actually know? Why do we now have view controller containment hierarchies as well as regular view hierarchies? How does it differ from a UIView
in this case? What does this all have to do with MVC?
What is a view controller?
To figure this out, we are going to start pulling code out of some hypothetical view controller and see what’s left at the end.
First we’ll move all that horrible view setup and layout code into a UIView
subclass. Done.
Then we’ll move all the core data and model code into some nice protocols/classes, which are injected into the view controller through public properties or an -init
method. Done.
We’ll move the table view delegate/datasource code into dedicated classes. Done.
Any miscellaneous framework code, url requests, etc. will also be pulled out behind nice interfaces, and our view controller will simply funnel data back and forth between the view and these interfaces. Done.
Finally, let’s delegate navigation events like certain button presses and cell taps to somebody else, so the view controller’s behaviour can be customized easily for use in different contexts. Done.
What are we left with at the end of all this?
We’re left with a class that:
- Lazily loads a view (by calling
-loadView
when the-view
property is accessed for the first time) - Can load its view from a NIB file in a convenient manner.
- Provides access to various events like
-viewDidAppear:
,-didRotateFromInterfaceOrientation:
, and-didReceiveMemoryWarning
. - Deals with state restoration to restore state between launches of your application.
- Handles storyboard segues to pass data to the view controller that is being transitioned to.
- Can provide accessibility information about the content it displays.
- Allows you to define which interface orientations are allowed when its view is at the top of the view hierarchy.
- Can be inserted into UIKit’s standard containers like
UINavigationController
andUITabBarController
(alsoUIWindow
), with a bunch of properties likenavigationItem
andtabBarItem
to customise its appearance in these. - Can be nested inside another view controller in a UIKit-safe way.
- Happens to be part of the responder chain, so can respond to and handle certain types of input events.
Wow. That’s a lot of stuff, and I’ve probably forgotten some things. It also sounds a hell of a lot like a view to me. The lines are starting to blur. It certainly doesn’t sound like a place for important business or application logic.
If you look at the actual interface for UIViewController
— the methods and properties it declares, it’s almost all view/presentation related. Go on, pop open Dash and take a look right now. I can only count 5 methods out of 86 that are not presentation related. These are -didReceiveMemoryWarning
and the 4 state restoration methods that came with iOS 6. Everything else is related to the view. And that’s not even including all the category methods that get added on by other UIKit headers.
An identity crisis
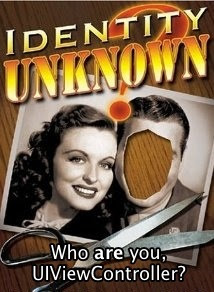
What if we continue to pull stuff out of UIViewController
?
-viewDidAppear:
and all the other view-related events would be much happier sitting on the UIView
class instead, for instance.
Lazy loading also seems to be a detail that belongs elsewhere. Don’t we just want something like this?
UILazyLoadedView *lazy = [[UILazyLoadedView alloc] initWithLoadViewBlock:^{ MyView *v = [[MyView alloc] init]; // restore view state return v; }];
Then we just change the UINavigationController
and other container classes to accept a UILazyLoadedView
where applicable. When our view needs to be displayed or loaded, UIKit can invoke the block and get a view returned to it. Any view state can be captured/restored in the block, making it work well when the view is unloaded/reloaded (prior to iOS 6 anyway).
Let’s look at the navigationItem
/tabBarItem
/etc. properties now.
These properties are used to customise the way the view controller appears in navigation controllers, tab bar controllers, etc. These customizations are always going to vary depending on the context in which the view controller is presented.
So do these properties really belong on the view controller? They’re actually part of the interfaces for the various container controllers that Apple gives us. But they’ve been “helpfully” tacked on to UIViewController
via categories. Is this really a good idea? Didn’t we already establish that a view controller should only know about the content it displays and manages?
If a view controller represents a screen of your app, why does it need to know the title of its back button when another screen is being displayed? Why does it need to have access to the icon image in its tab bar button? Shouldn’t that be managed by the class that set up the tab bar controller, or that pushed it onto the navigation controller?
When we consider the multitude of responsibilities a view controller already has, does it really need to deal with the specifics of all these container controllers as well?
Couldn’t we remove these category methods entirely and provide the information when we do the actual push? Something like this:
UILazyLoadedView *lazyView = /*...*/; UINavigationControllerItem *item = [[UINavigationControllerItem alloc] initWithLazyView:lazyView title:NSLocalizedString(@"foobar", nil) backButtonTitle:/*...*/ /* etc */]; [navController pushItem:item];
I don’t know. We’re getting dangerously close to the realm of pure fantasy here. But we need to think about these things to figure out exactly what a view controller is, and what it’s main roles should be. Everything we’ve seen so far seems to belong somewhere else.
The other thing view controllers can do is “view controller containment”. This can only be described as a complete (and unnecessary) mess.
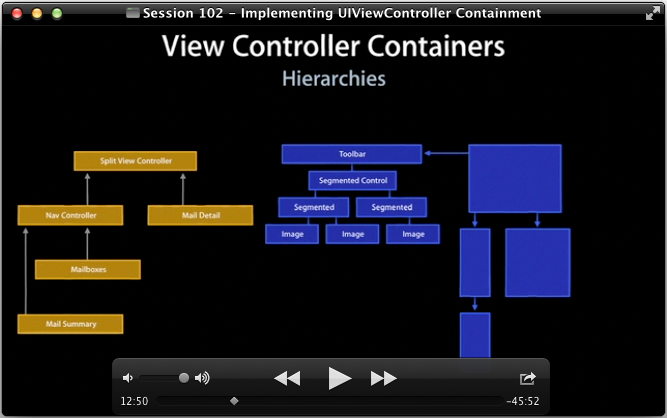
Because a view controller is just a wrapper around a view, people ended up needing to nest or compose view controller views in a single screen. But things like rotation events, -viewDidAppear:
etc. get all screwed up if you do this by manipulating the view hierarchy directly. This wouldn’t even be a problem if these methods were part of UIView
instead.
So to make this work nicely, Apple has given us the view controller containment APIs, with weird-ass requirements in the order you call the various willMoveToViewController/didMoveToViewController/addChildViewController/etc.
methods.
And now that we have this view controller containment, a view controller no longer represents an entire screen of content. It represents a portion of the screen and can be nested arbitrarily with other view controllers. So… doesn’t that just make it a view? A view with some additional UIKit details hacked on?
Somehow this all feels like a big mess to me. I’ve only covered a few items from UIViewController
’s interface, but it’s already starting to look like this weird “miscellaneous crap that UIKit needs” class with a lazy loaded view inside it. It’s a confused mess of responsibilities.
It also happens to contain the majority of our application code. This is a problem.
A black hole of bad design.
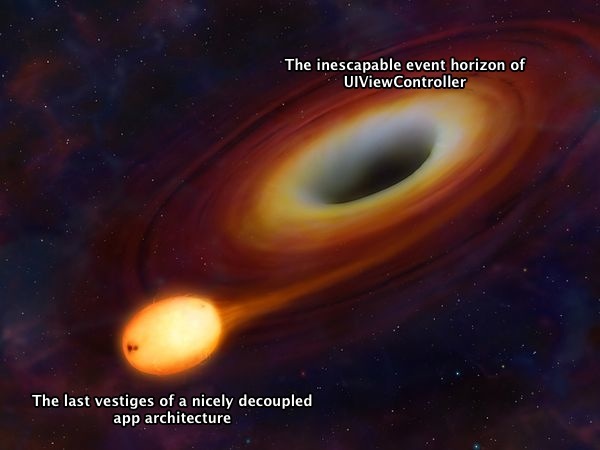
Let’s summarise the key points so far.
UIViewController
is the centre of the iOS world. Apple’s tools like Xcode and Interface Builder, and the workflow they encourage, put the view controller at the centre of everything. The default place to start when you’re adding new code or features is the view controller. When you add a new screen to your app, all you’re given is a UIViewController
subclass and a lot of helpful methods to shoot yourself in the foot.
Because of this, code and logic tend to gravitate towards the view controller. The view controller ends up being the delegate for a large number of different objects. It ends up doing view setup and layout, it ends up dealing with core data, table view datasource/delegate methods, and a huge number of other responsibilities.
Apple has tacked on a large number of “convenience” methods which let you directly access the enclosing containers like navigation controllers or tab bar controllers. This makes it very easy to couple the view controller to the specifics of your app’s navigation, and to the other view controllers it interacts with. Storyboards also contribute to this problem. This makes it difficult to reuse the view controller in other contexts or applications.
Even if you manage to keep your own interfaces/APIs nicely decoupled and separated into other classes, UIViewController
is the only place to deal with certain UIKit details, like: lazy-loading of views, configuring the display of tab bar items and navigation controller elements, view events like viewDidAppear
, rotation events, and view controller containment when you need to nest multiple view controllers inside one another.
If you have any kind of decision making logic in your view controller, it is completely coupled to the UIKit framework. As we’ll see in my next post, trying to get these things into a test harness is a disaster waiting to happen.
UIViewController
is just a detail of UIKit’s implementation. It’s more of a view than a controller, and certainly isn’t anywhere close to being a Controller in the classical MVC sense, despite what Apple tries to tell us:
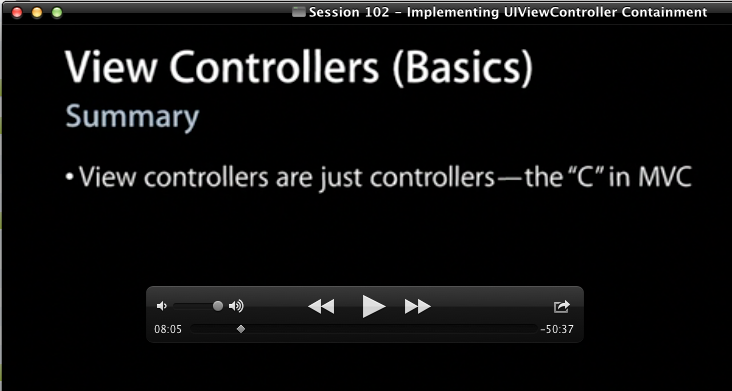
“Controllers” are supposed to be nice thin objects funnelling data and events between the model and the view. They shouldn’t contain details of a specific UI framework. iOS’ View controllers are nothing like this. They sit firmly in the presentation layer.
So why are we putting so much of our important application and workflow logic into our view controllers? I feel like the iOS developer community has forgotten everything we have learnt about good OOP design in the last 20 years or so. Remember the Single Responsibility Principle? One Reason To Change? Mixing application logic in amongst the spaghetti of UIKit APIs is just plain wrong.
Isn’t there a better way? Isn’t there an escape from this madness?
I’m happy to tell you that there is a better way.
I’m going to take you on a journey, Dear Reader. A journey of self-discovery and enlightenment. Or… something like that. We’ll figure it out as we go.
We’re going to develop a Clean iOS Architecture together, driven by TDD. We’re going to find out why so many people are doing iOS TDD wrong, and how to do it right. And we’re going to see some really nice benefits from this new approach.
Won’t you join me?
PS: I’m currently looking for new job opportunities, preferably in the UK. London would be ideal. Please contact me using the email address at the top of this page if you have any offers.